JavaScript To Haskell Converter
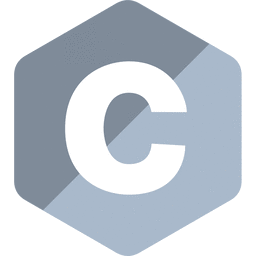
Other JavaScript Converters
What Is JavaScript To Haskell Converter?
An AI JavaScript to Haskell converter is a specialized online tool that leverages advanced technologies such as generative AI, machine learning, and natural language processing to facilitate the conversion of JavaScript code into Haskell language. This converter serves as a bridge between two fundamentally different programming environments, allowing developers to transition code seamlessly without the need to rewrite everything from scratch.
The conversion process involves three primary steps:
- Input: You provide the JavaScript code that requires conversion.
- Processing: The tool analyzes the provided code, employing its underlying algorithms to understand the structure and semantics of the JavaScript code. This stage is crucial as it involves mapping the JavaScript constructs to their Haskell equivalents, considering the differences in paradigms and data types.
- Output: The tool generates the converted code in Haskell, which is then presented for review and use.
How Is JavaScript Different From Haskell?
JavaScript and Haskell represent two distinct approaches to programming, each with its own strengths and weaknesses tailored to different types of projects. JavaScript, often hailed as the backbone of web development, is a dynamic and interpreted language. This means it can execute code directly in web browsers without needing to be compiled beforehand. Its flexibility is evident in its ability to support event-driven, functional, and imperative programming styles, making it suitable for a wide range of interactive applications. However, this dynamism can sometimes lead to challenges in managing code complexity, especially in larger applications.
In contrast, Haskell stands as a statically typed, purely functional programming language, which prioritizes immutability and treats functions as first-class citizens. This means that once you define a value in Haskell, it cannot be changed, leading to fewer unexpected behaviors. The transition from JavaScript to Haskell isn’t just about learning new syntax; it requires adopting a fundamentally different mindset around programming, focusing on safety, robustness, and mathematical precision.
Let’s look at some key distinctions between JavaScript and Haskell:
- Typing: JavaScript employs dynamic typing, allowing developers to write code without strict type definitions. Conversely, Haskell uses static typing, meaning types are checked at compile-time, which can help catch errors early in the development process.
- Execution Model: JavaScript is interpreted, enabling quick development and testing directly within browsers. Haskell, on the other hand, compiles code into native machine code, potentially increasing performance, particularly for computationally intensive tasks.
- Functional Capabilities: While both languages support functional programming, Haskell is purely functional, enforcing a style where functions have no side effects. JavaScript, meanwhile, combines imperative and functional techniques, which can lead to more flexible, but sometimes messier, code design.
Feature | JavaScript | Haskell |
---|---|---|
Typing | Dynamic | Static |
Execution | Interpreted | Compiled |
Paradigm | Multi-paradigm | Functional |
State Management | Mutable state | Immutable state |
Concurrency Model | Event-driven | Software Transactional Memory |
How Does Minary’s JavaScript To Haskell Converter Work?
The Minary JavaScript To Haskell converter operates through a straightforward yet effective process. First, you need to describe your task in detail in the designated input box on the left side of the generator. This description should provide enough context for the converter to produce accurate results based on your requirements. Whether you’re looking to convert simple functions or complex logic, clarity in your task description will enhance the outcome.
Once you fill out the details, click the “Generate” button. The system processes your input and promptly displays the generated Haskell code on the right side. You’ll find an easy-to-use interface that also features a “Copy” button at the bottom, allowing you to quickly transfer the generated code to your clipboard for further use.
Additionally, there are feedback vote buttons available for you to assess the quality of the generated code. Your input is valuable—it helps train and refine the JavaScript To Haskell converter, ensuring more precise outputs over time.
For example, suppose you describe a task like “Convert a function that calculates the factorial of a number from JavaScript to Haskell.” After clicking generate, you would see a neatly formatted Haskell equivalent of your factorial function, ready for implementation or further modification. With the conversion process being so seamless, leveraging the Minary JavaScript To Haskell converter can substantially streamline your coding workflow.
Examples Of Converted Code From JavaScript To Haskell
“Believe you can and you’re halfway there. -Theodore Roosevelt”,
“The only way to do great work is to love what you do. -Steve Jobs”,
“The future belongs to those who believe in the beauty of their dreams. -Eleanor Roosevelt”,
“Success is not final, failure is not fatal: It is the courage to continue that counts. -Winston S. Churchill”,
“What lies behind us and what lies before us are tiny matters compared to what lies within us. -Ralph Waldo Emerson”,
“Act as if what you do makes a difference. It does. -William James”,
“You are never too old to set another goal or to dream a new dream. -C.S. Lewis”,
“What’s important is to believe in yourself and know that you’re capable of achieving your dreams. -Unknown”
];
function getRandomQuote() {
const randomIndex = Math.floor(Math.random() * quotes.length);
return quotes[randomIndex];
}
console.log(getRandomQuote());
quotes =
[ “Believe you can and you’re halfway there. -Theodore Roosevelt”
, “The only way to do great work is to love what you do. -Steve Jobs”
, “The future belongs to those who believe in the beauty of their dreams. -Eleanor Roosevelt”
, “Success is not final, failure is not fatal: It is the courage to continue that counts. -Winston S. Churchill”
, “What lies behind us and what lies before us are tiny matters compared to what lies within us. -Ralph Waldo Emerson”
, “Act as if what you do makes a difference. It does. -William James”
, “You are never too old to set another goal or to dream a new dream. -C.S. Lewis”
, “What’s important is to believe in yourself and know that you’re capable of achieving your dreams. -Unknown”
]
getRandomQuote :: IO String
getRandomQuote = do
randomIndex <- randomRIO (0, length quotes – 1) return (quotes !! randomIndex) main :: IO () main = do quote <- getRandomQuote putStrLn quote
“The only way to do great work is to love what you do. – Steve Jobs”,
“Believe you can and you’re halfway there. – Theodore Roosevelt”,
“The only limit to our realization of tomorrow is our doubts of today. – Franklin D. Roosevelt”,
“Act as if what you do makes a difference. It does. – William James”,
“Success is not the key to happiness. Happiness is the key to success. – Albert Schweitzer”
];
function getRandomQuote() {
const randomIndex = Math.floor(Math.random() * quotes.length);
return quotes[randomIndex];
}
function displayQuote() {
const quote = getRandomQuote();
const currentDateTime = new Date().toLocaleString();
console.log(`Inspirational Quote: “${quote}”nDisplayed on: ${currentDateTime}`);
}
displayQuote();
quotes =
[ “The only way to do great work is to love what you do. – Steve Jobs”
, “Believe you can and you’re halfway there. – Theodore Roosevelt”
, “The only limit to our realization of tomorrow is our doubts of today. – Franklin D. Roosevelt”
, “Act as if what you do makes a difference. It does. – William James”
, “Success is not the key to happiness. Happiness is the key to success. – Albert Schweitzer”
]
getRandomQuote :: IO String
getRandomQuote = do
randomIndex <- randomRIO (0, length quotes – 1) return (quotes !! randomIndex) displayQuote :: IO () displayQuote = do quote <- getRandomQuote currentDateTime <- getCurrentTime >>= return . formatTime defaultTimeLocale “%c”
putStrLn $ “Inspirational Quote: “” ++ quote ++ “”””nDisplayed on: “” ++ currentDateTime